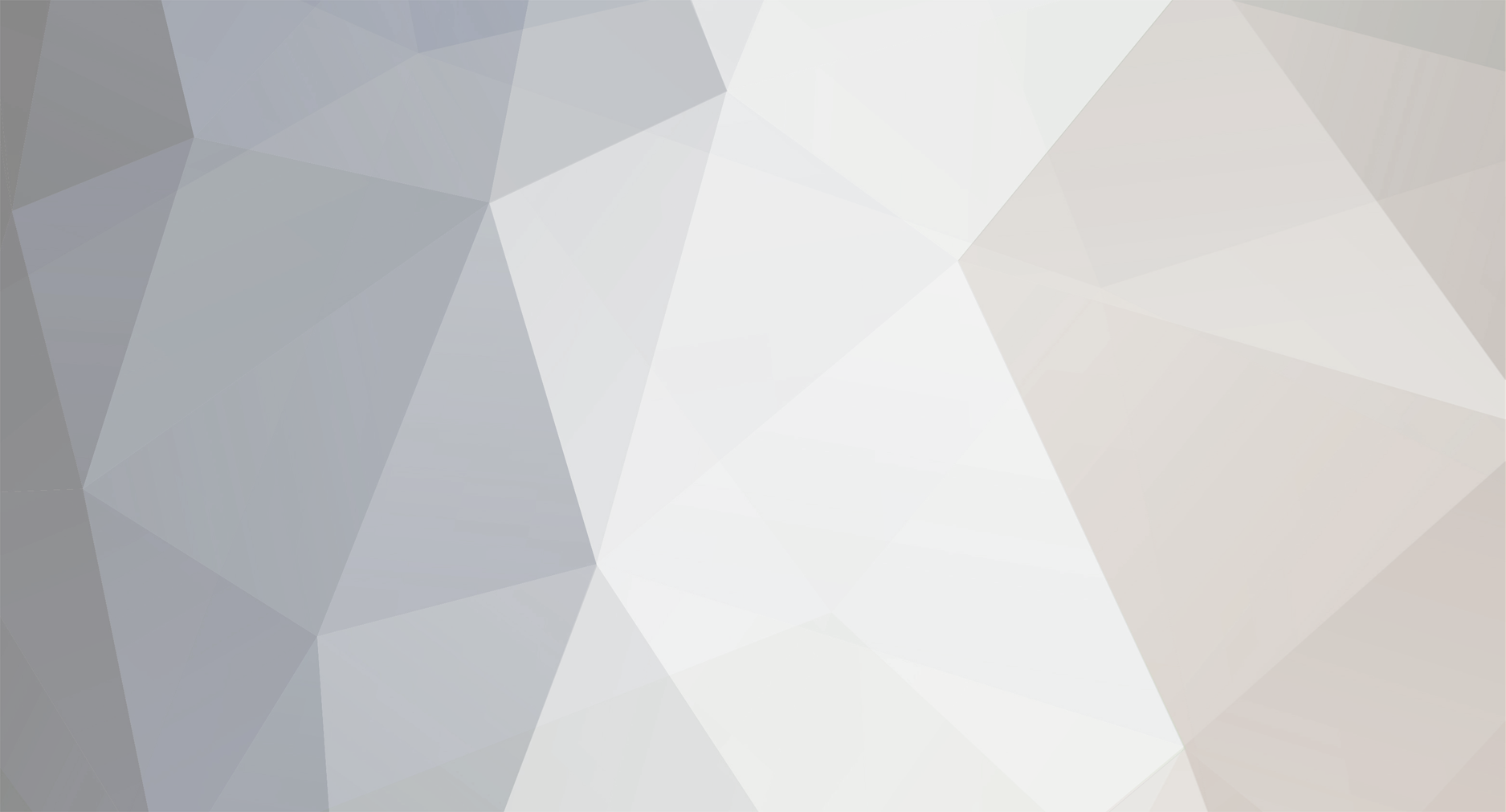
DannyR
Members-
Posts
4 -
Joined
-
Last visited
Profile Information
-
Gender
Male
-
Location
Everywhere
DannyR's Achievements
Newbie (1/14)
0
Reputation
-
HHN 21 | Take A Chance Clues & Answers
DannyR replied to King_K.'s topic in Halloween Horror Nights 21
Well, there are very few permutations so I'm not all that surprised you encountered a pattern, but it is still random. The image for the chest/door thing is static, and can be extracted from the game. It's shown below: There are actually a pile of rune symbols (more than 4) that appear in the game, but I didn't bother extracting all of those. Here is some pseudo-code derived from the AVM bytecode responsible for linking rune-keys to rune-slots. You can ignore the reference to 'door' in this case, as it looks like the devs copied/pasted some of the code from the key puzzle game. The source for the random feed is again Math.random(). private function _SetKeyDoorCombo() : void { var random_key_index:Number = NaN; var random_key:String = null; var runeKeyNumber:uint = 0; var rune_slot_index:uint = 1; while (rune_slot_index <= 4) { random_key_index = Math.random() * mKeyList.length >> 0; random_key = mKeyList.splice(random_key_index, 1)[0]; mInstance["mDoor" + rune_slot_index + "CorrectKey"] = MovieClip(mcContainer.getChildByName(random_key)); runeKeyNumber = uint(StringUtils.remove(String(random_key), "key")); mcContainer["door" + rune_slot_index].mcRune.gotoAndStop(runeKeyNumber); rune_slot_index = rune_slot_index + 1; } return; }// end function The AVM bytecode is below: private function _SetKeyDoorCombo() : void { _as3_getlocal <0> _as3_pushscope _as3_pushnan _as3_setlocal <2> _as3_pushnull _as3_coerce_s _as3_setlocal <3> _as3_pushbyte 0 _as3_convert_u _as3_setlocal <4> _as3_findpropstrict trace _as3_pushstring "---------------" _as3_callpropvoid trace(param count:1) _as3_findpropstrict trace _as3_pushstring "Certain Rune > Set Combo" _as3_callpropvoid trace(param count:1) _as3_pushbyte 1 _as3_convert_u _as3_setlocal <1> _as3_jump offset: 138[#83] #21 _as3_label _as3_getlex Math _as3_callproperty random(param count:0) _as3_getlex mKeyList _as3_getproperty length _as3_multiply _as3_pushbyte 0 _as3_rshift _as3_convert_d _as3_setlocal <2> _as3_getlex mKeyList _as3_getlocal <2> _as3_pushbyte 1 _as3_callproperty http://adobe.com/AS3/2006/builtin::splice(param count:2) _as3_pushbyte 0 _as3_getproperty {} _as3_coerce_s _as3_setlocal <3> _as3_getlex mInstance _as3_pushstring "mDoor" _as3_getlocal <1> _as3_add _as3_pushstring "CorrectKey" _as3_add _as3_findpropstrict flash.display::MovieClip _as3_getlex mcContainer _as3_getlocal <3> _as3_callproperty getChildByName(param count:1) _as3_callproperty flash.display::MovieClip(param count:1) _as3_setproperty {} _as3_findpropstrict uint _as3_getlex com.xstudiosinc.utils::StringUtils _as3_findpropstrict String _as3_getlocal <3> _as3_callproperty String(param count:1) _as3_pushstring "key" _as3_callproperty remove(param count:2) _as3_callproperty uint(param count:1) _as3_convert_u _as3_setlocal <4> _as3_getlex mcContainer _as3_pushstring "door" _as3_getlocal <1> _as3_add _as3_getproperty {} _as3_getproperty mcRune _as3_getlocal <4> _as3_callpropvoid gotoAndStop(param count:1) _as3_findpropstrict trace _as3_getlex mInstance _as3_pushstring "mDoor" _as3_getlocal <1> _as3_add _as3_pushstring "CorrectKey" _as3_add _as3_getproperty {} _as3_getproperty name _as3_callpropvoid trace(param count:1) _as3_getlocal <1> _as3_increment _as3_convert_u _as3_setlocal <1> #83 _as3_getlocal <1> _as3_pushbyte 4 _as3_ifle offset: -145[#21] _as3_findpropstrict trace _as3_pushstring "---------------" _as3_callpropvoid trace(param count:1) _as3_returnvoid }// end function -
HHN 21 | Take A Chance Clues & Answers
DannyR replied to King_K.'s topic in Halloween Horror Nights 21
Well, dunno if this qualifies as a spoiler or not, but the rune game entrance doesn't tell you anything at all about the rune sequence. The image on the box is static. The runes are randomized and paired to the slots. Another purely random game. The machine game is a bit more interesting than the rest in that it is indeed random, but it's more like a memory game. Probably the best of the four so far. -
HHN 21 | Take A Chance Clues & Answers
DannyR replied to King_K.'s topic in Halloween Horror Nights 21
What changes have been made so far? I was out of town for a week. I'm not sure what they were before, but I don't think they can make them any more random than they are right now. -
HHN 21 | Take A Chance Clues & Answers
DannyR replied to King_K.'s topic in Halloween Horror Nights 21
Hello! I'm a long time forum stalker that never registered before I saw this thread, and I thought I'd take some time to help shed some light on this game. First of all, I apologize of this destroys the 'fun' in the game for some of you, there are quite a few spoilers. Please stop reading if this is the case. :-) At some point in the future the game will present the user with up to 9 cards, the template is shown here: These cards will lead the user to one of seven 'houses', of which three are currently available (house00-house02). The layout of the cards on the screen is random (client side), and the color of the cards assigned to each house is determined by the server on each render. As you've certainly experienced, some cards have the potential to immediately cost tokens. Other's will lead directly to a game or to a 'pre-game' (as illustrated well by the graves before the key game). The games are enabled or disabled by the server, and this is returned to the client along with a list that determines the 'risk' associated with each game. If the game isn't mentioned in the list of available games it is presumed disabled by the client (as is currently the case with all but two of the games). Regarding 'Thekey', as you've almost certainly noticed there are two tombs hard-coded to lead to failure. This is what is called the 'pre-game', but it's not much of a game. This leads to the real game, which involves 5 keys and 3 doors. I've seen some of you speculate about patterns, sequences, etc, but unfortunately this isn't the case either. It is entirely based on a PRNG. To illustrate the point, the code responsible for mapping keys to doors when the game is started is shown below: private function _SetKeyDoorCombo() : void { _as3_getlocal <0> _as3_pushscope _as3_pushnan _as3_setlocal <1> _as3_pushnull _as3_coerce_s _as3_setlocal <3> _as3_pushundefined _as3_coerce_a _as3_setlocal <4> _as3_pushbyte 1 _as3_convert_u _as3_setlocal <2> _as3_jump offset: 99[#62] #15 _as3_label _as3_getlex Math _as3_callproperty random(param count:0) _as3_getlex mKeyList _as3_getproperty length _as3_multiply _as3_pushbyte 0 _as3_rshift _as3_convert_d _as3_setlocal <1> _as3_getlex mKeyList _as3_getlocal <1> _as3_pushbyte 1 _as3_callproperty http://adobe.com/AS3/2006/builtin::splice(param count:2) _as3_pushbyte 0 _as3_getproperty {} _as3_coerce_s _as3_setlocal <3> _as3_getlex mcContainer _as3_getlocal <3> _as3_callproperty getChildByName(param count:1) _as3_coerce_a _as3_setlocal <4> _as3_getlex myPath _as3_pushstring "mDoor" _as3_getlocal <2> _as3_add _as3_pushstring "CorrectKey" _as3_add _as3_findpropstrict flash.display::MovieClip _as3_getlocal <4> _as3_callproperty flash.display::MovieClip(param count:1) _as3_setproperty {} _as3_findpropstrict trace _as3_getlex myPath _as3_pushstring "mDoor" _as3_getlocal <2> _as3_add _as3_pushstring "CorrectKey" _as3_add _as3_getproperty {} _as3_getproperty name _as3_callpropvoid trace(param count:1) _as3_getlocal <2> _as3_increment _as3_convert_u _as3_setlocal <2> #62 _as3_getlocal <2> _as3_pushbyte 3 _as3_ifle offset: -106[#15] _as3_returnvoid }// end function This can be represented as: private function _SetKeyDoorCombo() : void { var random_index:Number = NaN; var child_index:String = null; var child_object:* = undefined; var door_number:uint = 1; while (door_number <= 3) { random_index = Math.random() * mKeyList.length >> 0; // Equiv to Math.floor(Math.random...) child_index = mKeyList.splice(random_index, 1)[0]; // pops a key off the keylist child_object = mcContainer.getChildByName(child_index); myPath["mDoor" + door_number + "CorrectKey"] = MovieClip(child_object); // links door number to a key door_number = door_number + 1; } return; } Or to put it simply, it's random. There are some strategies that can be used to be efficient about discovering the map between keys and doors, but in general your odds of succeeding with less than 3 errors are pretty low (something like 15-20% according to my horrible math skillz). The 'Channelsurfing' pre-game involves another simple choice between two buttons, one fails one moves you on to the actual game. The actual game is nine screens, three of which are 'bad'. The bad ones are positioned entirely randomly, as seen with the key/door combos. They instantiate all 9 elements of the screen list with 'good', and then mark 3 screens as 'bad', pseudocode below. while (screen_count <= 9) { mScreenList.push(new Screen(this, this.mcContainer["s" + screen_count])); MovieClip(this.mcContainer["s" + screen_count]).gotoAndStop(2); screen_count = screen_count + 1; } while (bad_screen_list.length < 3) { random_selected_screen = mScreenList[uint(Math.random() * mScreenList.length)]; if (bad_screen_list.indexOf(random_selected_screen) == -1) { random_selected_screen.isBad = true; bad_screen_list.push(random_selected_screen); } } The odds of winning the channel surfing game > the key game. In any event, shortly after hitting a failure or a success in any game your score is immediately pushed to the server along with a checksum. At this point in the game no score seems to unlock any additional content. Even if it somehow did unlock additional content, the swf files for the other houses and games are not yet present on the server so they would quite literally do nothing. Regards, Danny